Python - #1 - The Basics
1. Start with Commenting
Programmers write A LOT of code. They need to understand exactly what they have written, especially if they are working as part of a team or returning to code after working on other projects.
​
To help them understand what they have written, programmers use comments to annotate (explain) their code.
Task 1 - Create a new Python program and use # to write a comment that says your name and the date. Save the file as 1-Basics.py
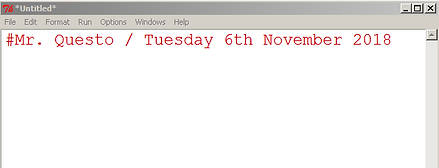
In Python, type the # symbol then your message to write a comment.
​
Comments are not printed when you run a program!
​
It is a good idea to start every program with a comment, so you know what the program is about.
2. Printing to the Screen
The most basic and common command you will use in Python is print.
​
Inside the print brackets, you can write a message within speech marks.
​
Your print command should turn purple - don't use any capital letters in Python unless it is inside speech marks!
Task 2 - Write a nice message by using the print command, brackets and speech marks.
​
Press F5 to run your program.


3. More Printing
You can write multiple print lines one after another to print on different lines.

Task 3 - Add two more print lines to your program. You can choose any message that you like.

4. New Lines
You can use the special command \n to start a new line. This allows you to write on multiple lines but only use one print line.
​
Use the backslash ( \ ) not the forward-slash ( / ).
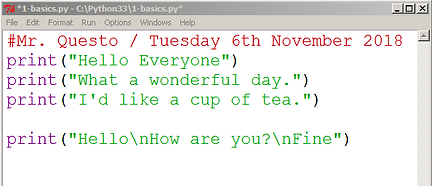
Task 4 - Use \n to write a 3 sentence conversation in only one line of code.

Challenge Programs
Use everything that you have learned on this page to help you create these programs...
​
Challenge Task 1 - Days of the Week
-
Create a new Python program. Save it as '1-Week.py'
-
Add a comment at the top with your name and the date.
-
Create a program that prints the days of the week, with each day on a new line.
​
-
BONUS: Try to use only one print line.
-
BONUS: Have no empty spaces at the start of each line.
​
When you run it, it should look like this:

Challenge Task 2 - Conversation
-
Create a new Python program. Save it as '1-Conversation.py'
-
Add a comment at the top with your name and the date.
-
Create a program that prints a 6-line conversation between two people. It is up to you what these two people are talking about.
​
-
BONUS: Try to use only one print line.
-
BONUS: Have no empty spaces at the start of each line.
​
When you run it, it could look something like this:
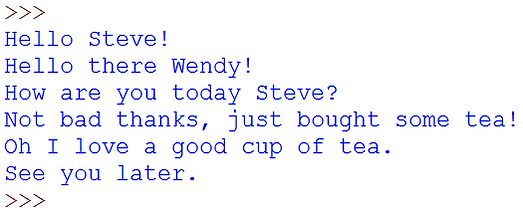