2.3: Additional Programming Techniques
Exam Board:
OCR
Specification:
J277
Array
An array is a static data structure that can hold a fixed number of data elements. Each data element must be of the same data type i.e. real, integer, string.
The elements in an array are identified by a number that indicates their position in the array. This number is known as the index. The first element in an array always has an index of 0.
​
You should know how to write pseudo code that manipulates arrays to traverse, add, remove and search data. The following steps uses Python as an example.
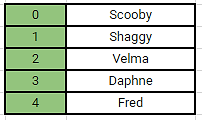
What
Traversing an Array
To traverse ('move through') an array a for loop can be used to display each data element in order.
'Inserting' a value
In an array the size is fixed so you cannot insert new values, but you can change the value of elements that already exist. Overwriting the fourth element (Daphne) with a new value (Laura) will change it from Daphne to Laura.
Example code for traversing:


Example code for inserting:


Output:
Output:
'Deleting' a value
In an array the size is fixed so you cannot delete values, but you can overwrite them as blank. Overwriting the second element (Shaggy) with a blank space makes it appear deleted.
Example code for deleting:

Output:

Searching an Array
For large arrays a for loop is needed to search through each element for a specific value. This example checks each name to see if it is equal to Velma.
Example code for searching:
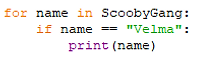
Output:

Two-Dimensional Array
Often the data we want to process comes in the form of a table. The data in a two dimensional array must still all be of the same data type, but can have multiple rows and columns.
​
The two-dimensional array to the right shows the characters from Scooby Doo along with their associated colour and their species.
​
Each value in the array is represented by an index still, but now the index has two values. For example [3] [0] is 'Daphne'. We measure row first, then column.
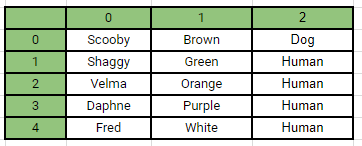

Searching a two-dimensional array:
To print a specific data element you can just use the index number like Daphne above. To search for a specific value you will need two for loops, one for the row and another for the values of each row.
The example to the right is looking for the value of 'Velma' and when it is round it prints the associated data from the whole row.
Example code for printing:

Output:

Example code for searching:

Output:

Records
Unlike arrays, records can store data of different data types.
Each record is made up of information about one person or thing.
Each piece of information in the record is called a field (each row name).
​
Records should have a key field - this is unique data that identifies each record. For example Student ID is a good key field for a record on students as no two students can have the same Student ID.
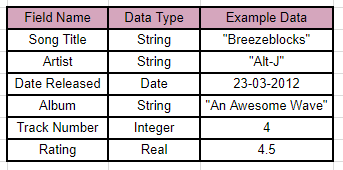
SQL
SQL (structured query language) is a language that can be used to search for data in a database.
The format of an SQL statement is:
SELECT field1, field2, field3…
FROM table
WHERE criteria
​
Example of an SQL statement using the Cars table:
​
SELECT Make, Colour
FROM Cars
WHERE Miles > 1000 AND Age > 8
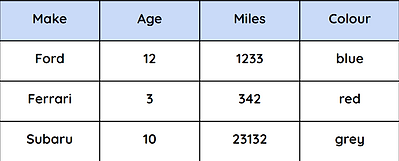
Cars table
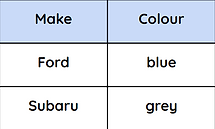
SQL uses wildcards which are symbols used to substitute characters.
The * symbol represents ALL fields.
​
Example:
​
SELECT *
FROM Cars
WHERE Colour = “blue”

Click the banner above to try a self-marking quiz (Google Form) on this topic.

Questo's Questions
2.3a - Additional Programming Techniques:
​
1. Describe the differences between a 1D array, 2D array and record. [3]
​
2. A one-dimensional array looks like this: TigerBreeds("Sumatran","Indian","Malayan,"Amur")
​
Write the code to:
-
a. Print the element with the index of 3. [2]
-
b. Change Indian to South China. [2]
-
c. Remove the Amur element. [2]
-
d. Search through the array for 'Malayan'. [2]
​
3a. Use the Cars table above to write the SQL statement to display the make and miles for cars that are grey OR blue. [3]
3b. Write an SQL statement to display all fields for cars that are 10 years old or less. [3]